Garbage Collection
Java garbage collection is the process by which java programs perform automatic memory management.
➡️ 메모리 자동 관리
When a Java program run on the JVM, objects are created in the heap space, which is a portion of memory dedicated to the program
➡️ 생성된 객체는 프로그램 메모리의 일부
Some objects will no longer be needed. To free up memory, the garbage collector discovers and removes these unused objects. Garbage collection is performed by a daemon thread called Garbage collector.
➡️ 각 객체는 수명을 가진다. 이러한 불필요한 객체를 가비지 컬렉터가 확인하여 제거해 준다.
the need for GC
In programming languages like C & C++, the programmer is responsible for destroying the objects.
If a programmer forgets to destroy unused objects, it will eventually leads to memory leaks and after a certain point there won’t be memory available to create new objects and the application fails with an out-of-memory error.
➡️ C & C++ 과 같은 언어에서는 개발자가 직접 사용하지 않는 객체의 메모리 공간을 관리해야 합니다.
➡️ 사용하지 않는 객체를 정리하지 않으면, 메모리 부족으로 인해 새로운 객체 생성 시 out-of-memory 에러가 발생할 수 있습니다.
In Java, GC happens automatically during the lifecycle of a program by freeing up memory, and therefore avoiding memory leaks.
➡️ Java에서는 프로그램 실행동안 자동으로 GC가 메모리 공간을 관리해 줍니다. 이를 통해 memory leak 문제를 방지할 수 있습니다.
Object state: Dead vs Alive
Over the lifetime of a Java application, new objects are created and released and at any point in time the heap memory consists of 2 types of objects: Dead and Alive.
➡️ 새로운 객체는 생성되고 해제됩니다. 여러 시점에 따라 Heap memory는 2 가지 형태의 객체가 구성됩니다.
Alive objects are being used and referenced from the applciation. Dead objects are no longer used or referenced from the application.
GC detects these objects and deletes them to free up the memory.
The object will not become a candidate for gc until all references to it are discarded.
➡️ Alive와 Dead 상태의 차이는 다른 곳에서 참조되어 사용되고 있느냐이다.
➡️ 객체에 대한 참조가 남아있으면 해당 객체는 gc 처리 대상이 아니다.
➡️ 즉, GC는 “Object’s Reference”를 기준으로 메모리에서 제거할 객체를 선택합니다.
GC : 3 basic steps
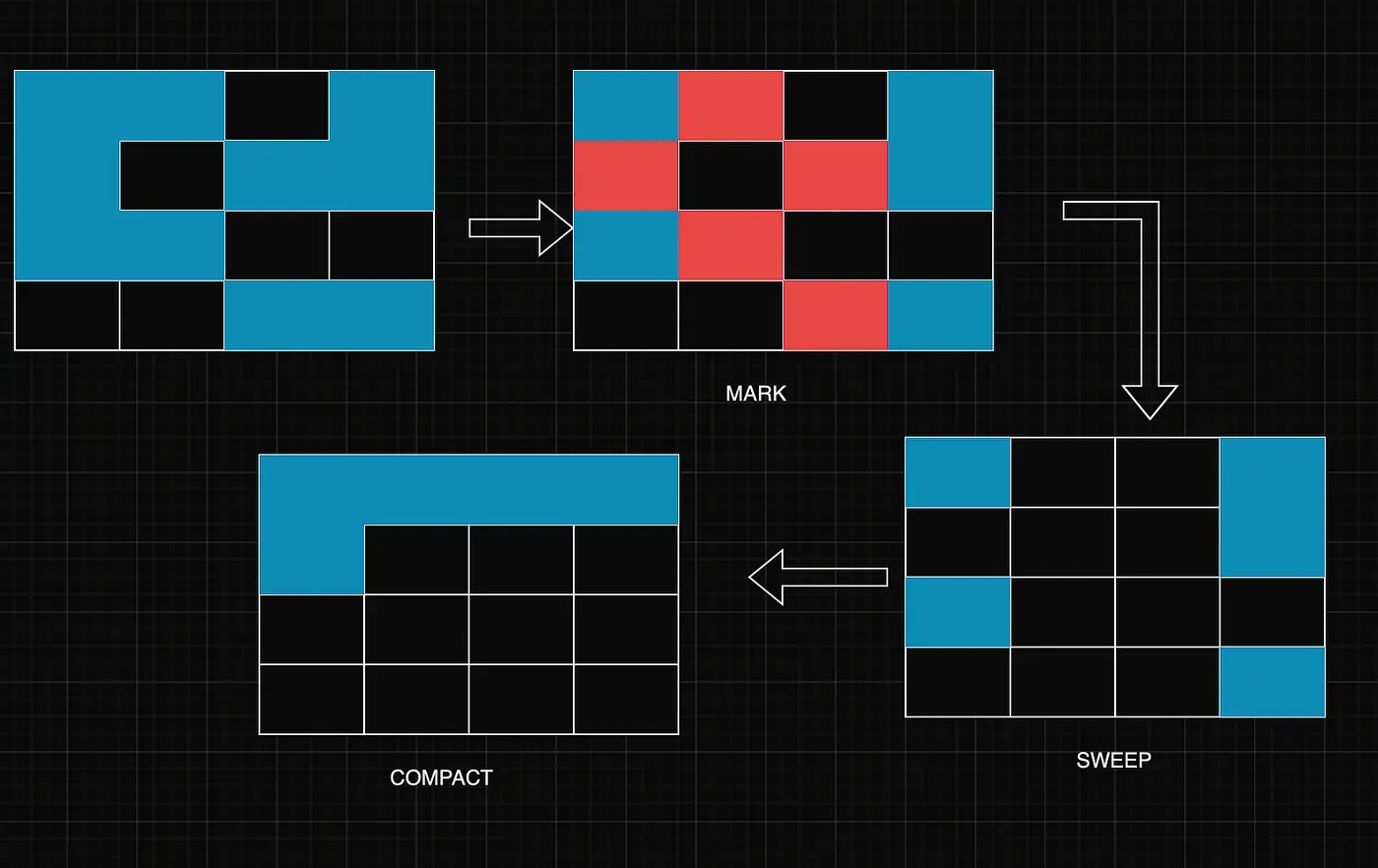
Mark
- Marking objects as alive, by traversing the object graph GC identifies all the alive objects in memory.
- The objects that are reachable by GC are marked as alive and objects that are not reachable are considered as eligible for GC
➡️ 마킹 작업은 가비지 컬렉터가 모든 객체를 탐색하며 reachable 한지 확인하여, 상태를 구분합니다.
➡️ unreachable 한 객체는 더 이상 참조가 되지 않은 객체이므로, GC가 제거해야 할 객체 대상이 됩니다.
Reachable 객체란
- GC Root에서 참조를 따라가며 접근할 수 있는 객체
- 즉, 참조되고 있는(사용되고 있는) 객체를 의미합니다.
Unreachable 객체란
- 참조가 끊어진 객체로 더 이상 사용되지 않는 Garbage로 간주되는 객체
- GC는 해당 객체를 Dead 상태로 간주하여 메모리를 회수합니다.
Sweep
- The second step is sweeping dead objects
- After identifying alive and dead objects, GC will free the memory which contains the dead objects.
➡️ Sweep 단계는 Dead Object의 메모리를 회수하는 단계입니다.
Compact
- During the sweep stage, the dead objects that were removed may not be next to each other which may result in memory fragementaion.
- Compact phase ensures in arranging the objects into the contiguous blocks at the start of the heap
➡️ 제거된 객체는 메모리 공간 상에서 순서대로 배치되지 않아, 메모리 조각이 발생할 수 있다.
➡️ Compact 단계에서 heap의 시작부터 객체들을 순서대록 배치시켜 줍니다.
Compact 단계가 필요한 이유
[메모리 단편화(Fragmentation) 감소 → 새로운 객체 할당 속도 및 메모리 활용도 증진]
- GC가 가비지 객체를 제거하면, 메모리 조각이 발생할 수 있습니다. 이로 인해 새로운 객체를 할당할 때, 메모리에 빈 공간이 있어도 연속된 큰 공간이 없으면 할당이 어려운 문제가 발생합니다.
- Compact 단계에서 Alive Object를 연속된 메모리 블록으로 이동하여 빈 공간을 한 곳에 모아줍니다.
GC: frequently mark and compact
Most of the objects are short lived and running mark and compact steps frequently on all the objects in the heap would be inefficient and time consuming.
In order to solve this, Java GC implements a generational garbage collection strategy that categorize objects by age.
➡️ 대부분 객체는 생명 주기가 짧아 mark & compact 작업이 빈번하게 발생한다. 이는 많은 비용이 들어 비효율적이다.
➡️ 이를 해결하기 위해 Java GC는 age(생성 시간)을 기준으로 “세대적 가비지 컬렉션 전략”을 수행합니다.
Generational GC
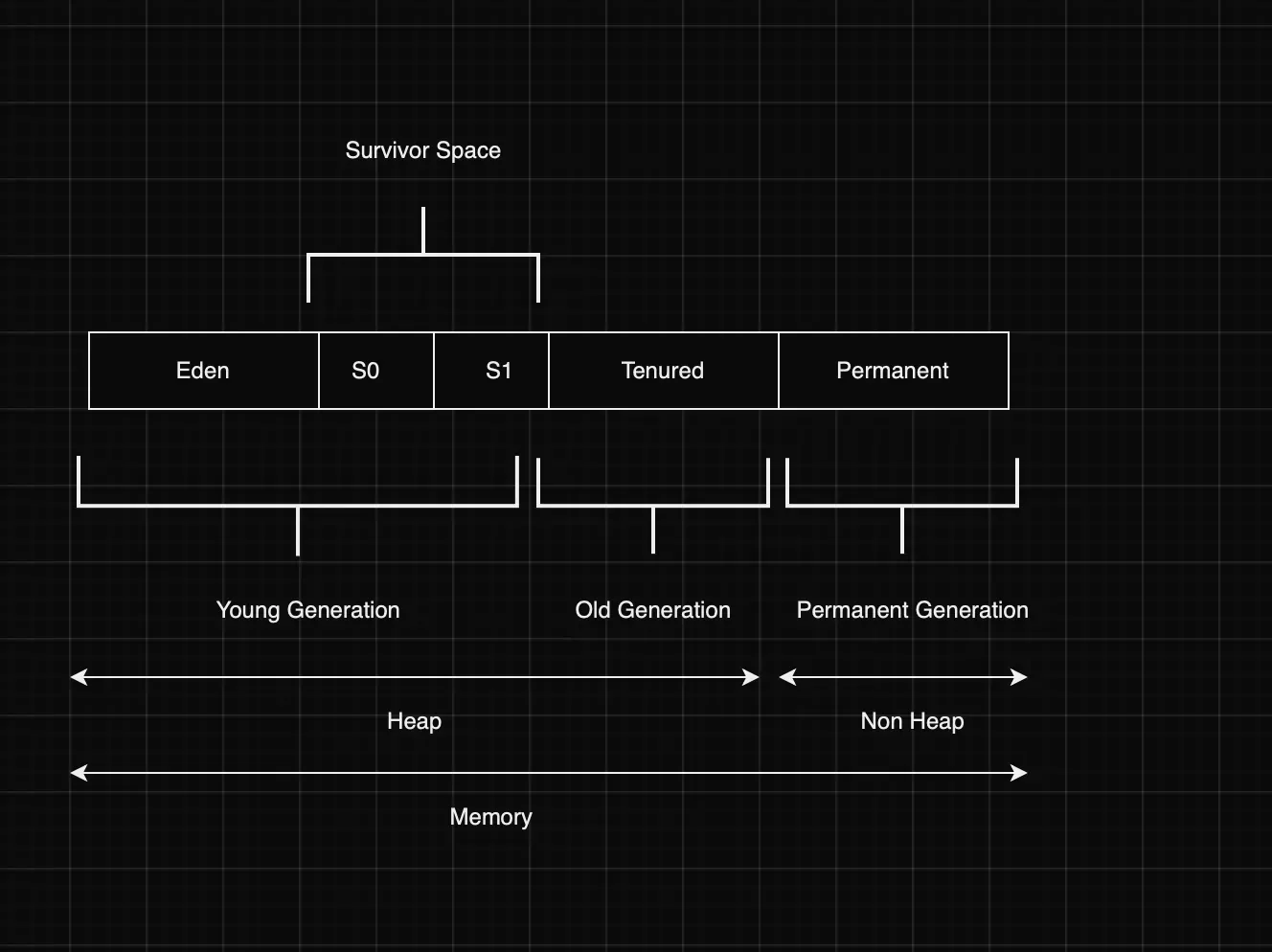
There are 3 different classification of objects by garbage collector.
Young, Old, Permanet Generation(Java 8 이후로 관리 X)
Youn Generation
- All the newly created objects start in young generation, which is subdivided into Eden space and two Survival spaces.
- When objects are garbage collected from the young generation, it is a minor garbage collection event.
Flow in youn generation
- objects are allocated in Eden space, while both survival spaces are empty.
- JVM performs a minor GC when Eden space is filled with objects (either dead or alive). Once the dead objects are removed, all the alive objects are now moved from Eden space to S0. Now, Eden and S1 are empty.
- When the Eden space is again filled with objects, another minor GC is performed and removes all the dead objects. This time, the alive objects from Eden space and S0 are moved to S1. Now, Eden and S0 are empty. At any given point of time one of the survivor spaces are empty.
- When the surviving objects reach a certain threshold of moving around the survivor space, they are moved to Old Generation.
1 단계 : 생성된 객체는 모두 Eden space에 할당된다.
2 단계 : Dead Object가 제거되면, 모든 Alive Object는 S0으로 이동합니다.
3 단계 : Dead Object가 또 제거되고, S0에 객체가 존재하는 경우 모든 Alive object와 S0 객체들이 S1로 이동합니다.
4 단계 : Survivor space로 이동해야 하는 특정 임계값에 도달하면 모두 Old space로 이동합니다.
Old Generation
- Eventually, the GC moves the long-lived objects from young generation to old generation.
- When objects are garbage collected from old generation, then it is a major garbage collection event.
- A full garbage collection cleans up both young and old generations.
Permanent Generation
- JVM stored the meta data such as classes and methods in the permanent generation.
➡️ 고정된 크기로 인해 메모리 관리가 어려웠음
MetaSpace
Java 8부터 Permanent Generation → MetaSpace로 대체됐다.
JVM의 Heap 메모리가 아닌, 운영체제에서 직접 할당하는 메모리 공간에 클래스 정보, 메서드 정보 등이 저장됩니다.
- OS가 제공하는 Native 메모리를 통해 동적 할당이 가능합니다.
- GC를 통해 간접적으로 Class Metadata를 정리할 수 있습니다.
- GC가 사용하지 않는 클래스를 제거하면, MetaSpace의 메모리가 줄어들어 Natvie 메모리가 해제될 수 있습니다.
참고자료
https://medium.com/@rakeshrdy8/garbage-collection-in-java-explained-bbebdc5f75ac
Garbage Collection in Java explained
“Java garbage collection is the process by which java programs perform automatic memory management”. JVM (Java Virtual Machine) can run…
medium.com
'JAVA' 카테고리의 다른 글
Java 23 ZGC 성능 개선 이해하기 – G1 GC와의 차이점 분석 (0) | 2025.04.05 |
---|---|
Java 객체 비교: equals()와 hashCode()로 보는 물리적 vs 논리적 동등성 (0) | 2025.03.31 |
Java 버전별 변화 및 주요 기능 - java 8, 11, 17, 21 (0) | 2025.03.14 |
Java Stream map vs flatMap 차이점과 활용법 (kotlin) (0) | 2025.03.04 |
Java 21 가상 스레드란? 기존 OS 스레드와의 차이점 정리 (0) | 2025.02.18 |
Garbage Collection
Java garbage collection is the process by which java programs perform automatic memory management.
➡️ 메모리 자동 관리
When a Java program run on the JVM, objects are created in the heap space, which is a portion of memory dedicated to the program
➡️ 생성된 객체는 프로그램 메모리의 일부
Some objects will no longer be needed. To free up memory, the garbage collector discovers and removes these unused objects. Garbage collection is performed by a daemon thread called Garbage collector.
➡️ 각 객체는 수명을 가진다. 이러한 불필요한 객체를 가비지 컬렉터가 확인하여 제거해 준다.
the need for GC
In programming languages like C & C++, the programmer is responsible for destroying the objects.
If a programmer forgets to destroy unused objects, it will eventually leads to memory leaks and after a certain point there won’t be memory available to create new objects and the application fails with an out-of-memory error.
➡️ C & C++ 과 같은 언어에서는 개발자가 직접 사용하지 않는 객체의 메모리 공간을 관리해야 합니다.
➡️ 사용하지 않는 객체를 정리하지 않으면, 메모리 부족으로 인해 새로운 객체 생성 시 out-of-memory 에러가 발생할 수 있습니다.
In Java, GC happens automatically during the lifecycle of a program by freeing up memory, and therefore avoiding memory leaks.
➡️ Java에서는 프로그램 실행동안 자동으로 GC가 메모리 공간을 관리해 줍니다. 이를 통해 memory leak 문제를 방지할 수 있습니다.
Object state: Dead vs Alive
Over the lifetime of a Java application, new objects are created and released and at any point in time the heap memory consists of 2 types of objects: Dead and Alive.
➡️ 새로운 객체는 생성되고 해제됩니다. 여러 시점에 따라 Heap memory는 2 가지 형태의 객체가 구성됩니다.
Alive objects are being used and referenced from the applciation. Dead objects are no longer used or referenced from the application.
GC detects these objects and deletes them to free up the memory.
The object will not become a candidate for gc until all references to it are discarded.
➡️ Alive와 Dead 상태의 차이는 다른 곳에서 참조되어 사용되고 있느냐이다.
➡️ 객체에 대한 참조가 남아있으면 해당 객체는 gc 처리 대상이 아니다.
➡️ 즉, GC는 “Object’s Reference”를 기준으로 메모리에서 제거할 객체를 선택합니다.
GC : 3 basic steps
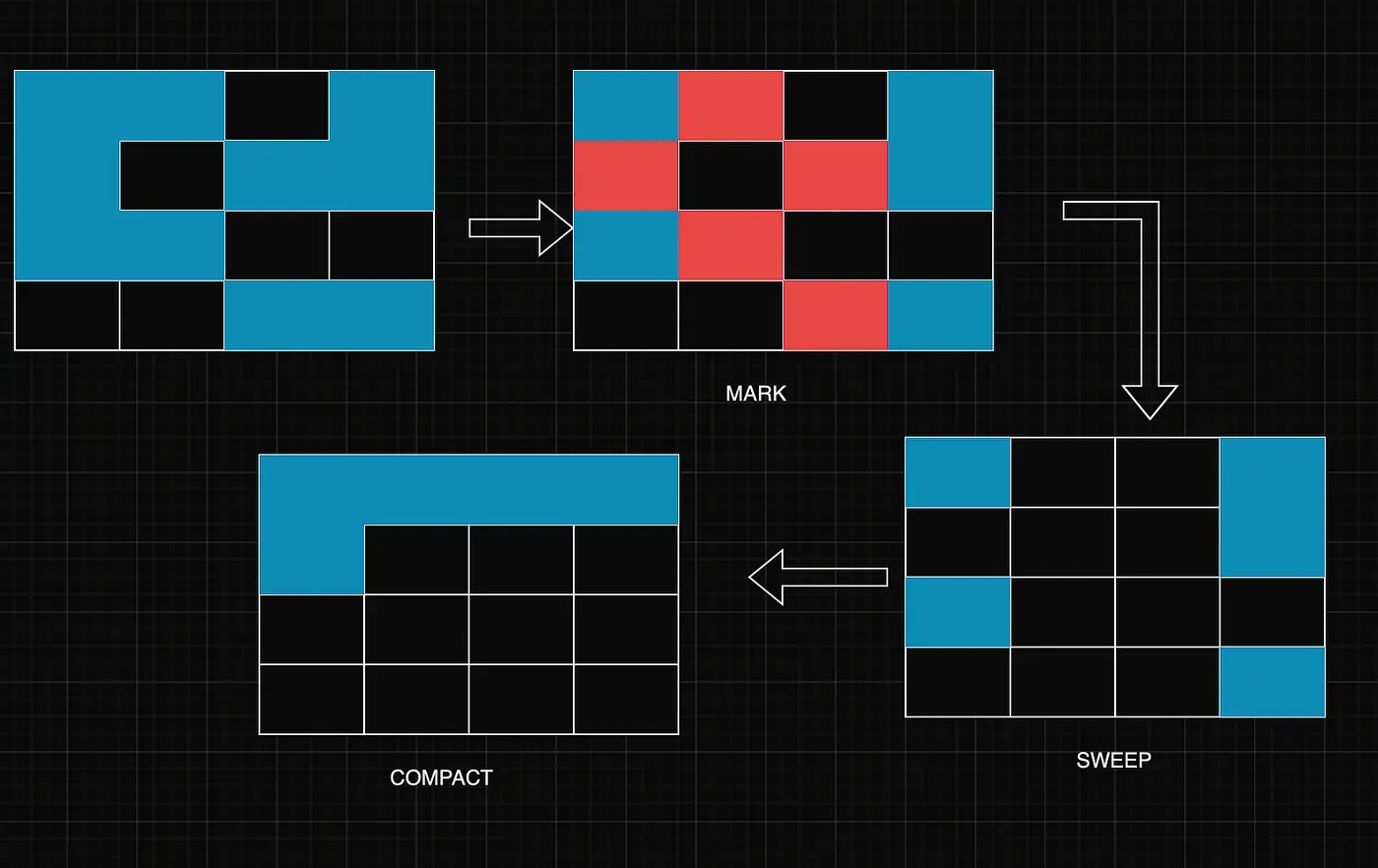
Mark
- Marking objects as alive, by traversing the object graph GC identifies all the alive objects in memory.
- The objects that are reachable by GC are marked as alive and objects that are not reachable are considered as eligible for GC
➡️ 마킹 작업은 가비지 컬렉터가 모든 객체를 탐색하며 reachable 한지 확인하여, 상태를 구분합니다.
➡️ unreachable 한 객체는 더 이상 참조가 되지 않은 객체이므로, GC가 제거해야 할 객체 대상이 됩니다.
Reachable 객체란
- GC Root에서 참조를 따라가며 접근할 수 있는 객체
- 즉, 참조되고 있는(사용되고 있는) 객체를 의미합니다.
Unreachable 객체란
- 참조가 끊어진 객체로 더 이상 사용되지 않는 Garbage로 간주되는 객체
- GC는 해당 객체를 Dead 상태로 간주하여 메모리를 회수합니다.
Sweep
- The second step is sweeping dead objects
- After identifying alive and dead objects, GC will free the memory which contains the dead objects.
➡️ Sweep 단계는 Dead Object의 메모리를 회수하는 단계입니다.
Compact
- During the sweep stage, the dead objects that were removed may not be next to each other which may result in memory fragementaion.
- Compact phase ensures in arranging the objects into the contiguous blocks at the start of the heap
➡️ 제거된 객체는 메모리 공간 상에서 순서대로 배치되지 않아, 메모리 조각이 발생할 수 있다.
➡️ Compact 단계에서 heap의 시작부터 객체들을 순서대록 배치시켜 줍니다.
Compact 단계가 필요한 이유
[메모리 단편화(Fragmentation) 감소 → 새로운 객체 할당 속도 및 메모리 활용도 증진]
- GC가 가비지 객체를 제거하면, 메모리 조각이 발생할 수 있습니다. 이로 인해 새로운 객체를 할당할 때, 메모리에 빈 공간이 있어도 연속된 큰 공간이 없으면 할당이 어려운 문제가 발생합니다.
- Compact 단계에서 Alive Object를 연속된 메모리 블록으로 이동하여 빈 공간을 한 곳에 모아줍니다.
GC: frequently mark and compact
Most of the objects are short lived and running mark and compact steps frequently on all the objects in the heap would be inefficient and time consuming.
In order to solve this, Java GC implements a generational garbage collection strategy that categorize objects by age.
➡️ 대부분 객체는 생명 주기가 짧아 mark & compact 작업이 빈번하게 발생한다. 이는 많은 비용이 들어 비효율적이다.
➡️ 이를 해결하기 위해 Java GC는 age(생성 시간)을 기준으로 “세대적 가비지 컬렉션 전략”을 수행합니다.
Generational GC
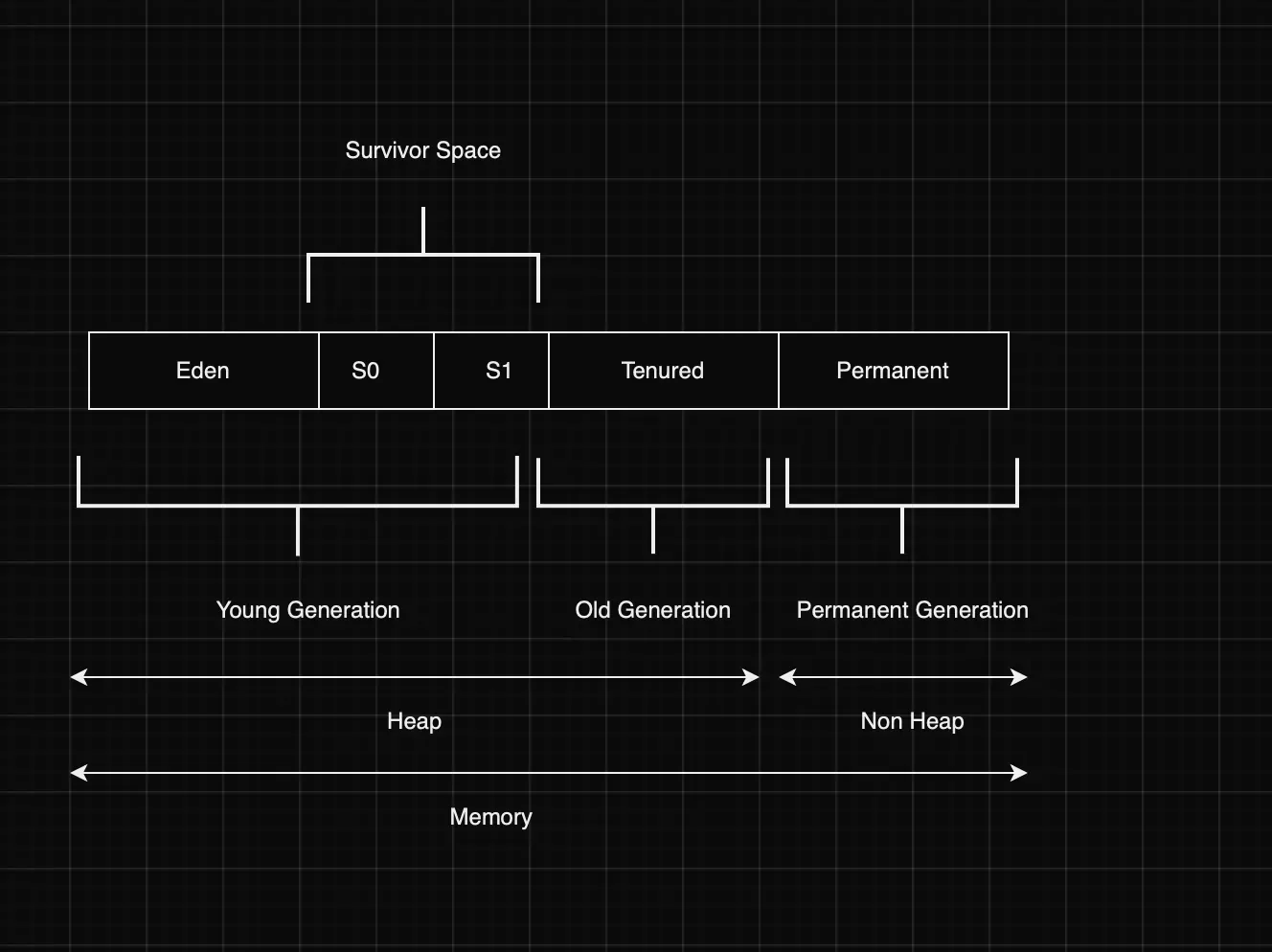
There are 3 different classification of objects by garbage collector.
Young, Old, Permanet Generation(Java 8 이후로 관리 X)
Youn Generation
- All the newly created objects start in young generation, which is subdivided into Eden space and two Survival spaces.
- When objects are garbage collected from the young generation, it is a minor garbage collection event.
Flow in youn generation
- objects are allocated in Eden space, while both survival spaces are empty.
- JVM performs a minor GC when Eden space is filled with objects (either dead or alive). Once the dead objects are removed, all the alive objects are now moved from Eden space to S0. Now, Eden and S1 are empty.
- When the Eden space is again filled with objects, another minor GC is performed and removes all the dead objects. This time, the alive objects from Eden space and S0 are moved to S1. Now, Eden and S0 are empty. At any given point of time one of the survivor spaces are empty.
- When the surviving objects reach a certain threshold of moving around the survivor space, they are moved to Old Generation.
1 단계 : 생성된 객체는 모두 Eden space에 할당된다.
2 단계 : Dead Object가 제거되면, 모든 Alive Object는 S0으로 이동합니다.
3 단계 : Dead Object가 또 제거되고, S0에 객체가 존재하는 경우 모든 Alive object와 S0 객체들이 S1로 이동합니다.
4 단계 : Survivor space로 이동해야 하는 특정 임계값에 도달하면 모두 Old space로 이동합니다.
Old Generation
- Eventually, the GC moves the long-lived objects from young generation to old generation.
- When objects are garbage collected from old generation, then it is a major garbage collection event.
- A full garbage collection cleans up both young and old generations.
Permanent Generation
- JVM stored the meta data such as classes and methods in the permanent generation.
➡️ 고정된 크기로 인해 메모리 관리가 어려웠음
MetaSpace
Java 8부터 Permanent Generation → MetaSpace로 대체됐다.
JVM의 Heap 메모리가 아닌, 운영체제에서 직접 할당하는 메모리 공간에 클래스 정보, 메서드 정보 등이 저장됩니다.
- OS가 제공하는 Native 메모리를 통해 동적 할당이 가능합니다.
- GC를 통해 간접적으로 Class Metadata를 정리할 수 있습니다.
- GC가 사용하지 않는 클래스를 제거하면, MetaSpace의 메모리가 줄어들어 Natvie 메모리가 해제될 수 있습니다.
참고자료
https://medium.com/@rakeshrdy8/garbage-collection-in-java-explained-bbebdc5f75ac
Garbage Collection in Java explained
“Java garbage collection is the process by which java programs perform automatic memory management”. JVM (Java Virtual Machine) can run…
medium.com
'JAVA' 카테고리의 다른 글
Java 23 ZGC 성능 개선 이해하기 – G1 GC와의 차이점 분석 (0) | 2025.04.05 |
---|---|
Java 객체 비교: equals()와 hashCode()로 보는 물리적 vs 논리적 동등성 (0) | 2025.03.31 |
Java 버전별 변화 및 주요 기능 - java 8, 11, 17, 21 (0) | 2025.03.14 |
Java Stream map vs flatMap 차이점과 활용법 (kotlin) (0) | 2025.03.04 |
Java 21 가상 스레드란? 기존 OS 스레드와의 차이점 정리 (0) | 2025.02.18 |