Sigleton Scope
the container creates a single instance of that bean
all requests for that bean name will return the same object. Any modifications to the object will be reflected in all references to the bean. This scope is the default value if no other scope is specified.
Sigleton Scope인 경우 빈에 대한 모든 요청은 같은 객체를 반환합니다.
객체에 대한 어떤 변경이 있는 경우 빈을 참조하는 다른 곳에도 반영이 됩니다.
즉, 싱글톤 방식으로 빈을 관리합니다.
@Bean
@Scope("singleton")
public Person personSingleton() {
return new Person();
}
Prototype Scope
A bean with the prototype scope will return a different instance every time it is requested from the container.
Prototype Scope 같은 경우에는 요청마다 다른 빈 인스턴스를 반환합니다.
즉, prototype scope 같은 경우는 하나의 틀을 가지고 요청마다 새로운 인스턴스를 생성하고 반환하여 사용하는 방식입니다.
@Bean
@Scope("prototype")
public Person personPrototype() {
return new Person();
}
Singleton & Prototype Scopre 테스트 코드
@Test
void testScope() {
AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(
SingletonPerson.class, PrototypePerson.class);
SingletonPerson bean1 = context.getBean(SingletonPerson.class);
SingletonPerson bean2 = context.getBean(SingletonPerson.class);
assertThat(bean1).isEqualTo(bean2);
PrototypePerson bean3 = context.getBean(PrototypePerson.class);
PrototypePerson bean4 = context.getBean(PrototypePerson.class);
assertThat(bean3).isNotEqualTo(bean4);
}
@Scope
static class SingletonPerson {
private String name;
}
@Scope("prototype")
static class PrototypePerson {
private String name;
}
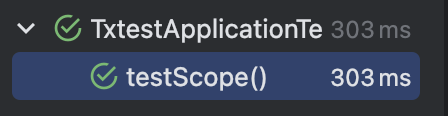
Web Aware Scope
The request scope creates a bean instance for a single HTTP request, while the session scope creates a bean instance for an HTTP Session.
테스트를 위한 Controller 코드
@Controller
@RequiredArgsConstructor
public class ScopeController {
private final ObjectProvider<MyLogger> myLoggerProvider;
private final LogService logService;
@GetMapping("/log-demo")
public String logDemo(HttpServletRequest request) throws InterruptedException {
final String requestURL = request.getRequestURL().toString();
final MyLogger myLogger = myLoggerProvider.getObject();
myLogger.setRequestUrl(requestURL);
myLogger.log("request scope test");
Thread.sleep(1000);
return "OK";
}
}
Request Scope
The ProxyMode attribute is necessary because at the moment of the instantion of the web application context, there is no active request.
Spring creates a proxy to be injected as a dependecy, and instantiates the target bean when it is needed in a request.
프록시 모드는 요청이 없는 경우 웹 애플리케이션 컨텍스트의 인스턴스화 상황 때문에 필요합니다.
요청이 생길 때 타겟 빈이 인스턴스화되어 사용됩니다.
Request Scope는 HTTP 요청마다 새로운 빈을 생성하여 사용합니다.
@Component
@Setter
@Scope("request")
public class MyLogger {
private String uuid;
private String requestUrl;
public void log(String msg) {
System.out.println("[" + uuid + "]" + "[" + requestUrl + "]" + msg);
}
@PostConstruct
public void init() {
uuid = UUID.randomUUID().toString();
System.out.println("[" + uuid + "] request scope bean created : " + this);
}
@PreDestroy
public void close() {
System.out.println("[" + uuid + "] request scope bean closed : " + this);
}
}

Session Scope
we need to run two requests in order to show that the value of the message field is the same for the session.
Session Sope 같은 경우는 하나의 세션 연결동안 생성한 빈을 세션 연결이 끊길 때까지 사용합니다.
@Component
@Setter
@Scope("session")
public class MyLogger {
private String uuid;
private String requestUrl;
public void log(String msg) {
System.out.println("[" + uuid + "]" + "[" + requestUrl + "]" + msg);
}
@PostConstruct
public void init() {
uuid = UUID.randomUUID().toString();
System.out.println("[" + uuid + "] request scope bean created : " + this);
}
@PreDestroy
public void close() {
System.out.println("[" + uuid + "] request scope bean closed : " + this);
}
}
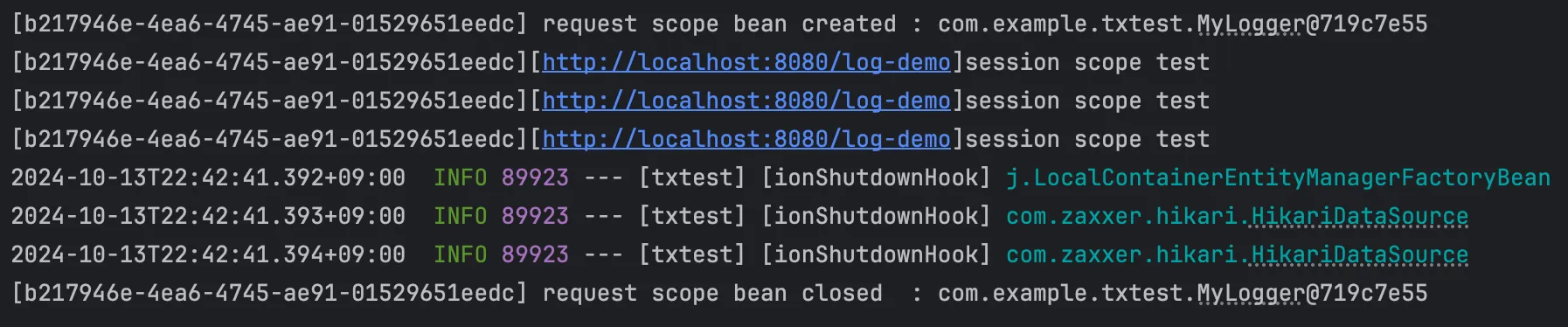
참고자료
Quick Guide to Spring Bean Scopes | Baeldung
Bean Scopes :: Spring Framework
7. [스프링 핵심 원리 - 기본편] 빈 스코프(Bean Scope)
'Spring Framework > Spring' 카테고리의 다른 글
Spring @RequestParam 페이징 정보 처리하기 - Pageable, @PageableDefault, sort (0) | 2024.10.19 |
---|---|
Spring StdSerializer, @JsonSerializer 커스텀 직렬화 처리 방법 (1) | 2024.10.16 |
스프링 FactoryBean: 생성자 주입과 필드 주입 시 프록시 객체의 동작 차이 (0) | 2024.10.12 |
Spring AOP 개념 정리 및 Aspect 적용 방법 - annotation 활용 (1) | 2024.10.10 |
[Spring] 스프링 FactoryBean 이해하기 - Custom Bean 생성방법 (2) | 2024.10.09 |
Sigleton Scope
the container creates a single instance of that bean
all requests for that bean name will return the same object. Any modifications to the object will be reflected in all references to the bean. This scope is the default value if no other scope is specified.
Sigleton Scope인 경우 빈에 대한 모든 요청은 같은 객체를 반환합니다.
객체에 대한 어떤 변경이 있는 경우 빈을 참조하는 다른 곳에도 반영이 됩니다.
즉, 싱글톤 방식으로 빈을 관리합니다.
@Bean
@Scope("singleton")
public Person personSingleton() {
return new Person();
}
Prototype Scope
A bean with the prototype scope will return a different instance every time it is requested from the container.
Prototype Scope 같은 경우에는 요청마다 다른 빈 인스턴스를 반환합니다.
즉, prototype scope 같은 경우는 하나의 틀을 가지고 요청마다 새로운 인스턴스를 생성하고 반환하여 사용하는 방식입니다.
@Bean
@Scope("prototype")
public Person personPrototype() {
return new Person();
}
Singleton & Prototype Scopre 테스트 코드
@Test
void testScope() {
AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(
SingletonPerson.class, PrototypePerson.class);
SingletonPerson bean1 = context.getBean(SingletonPerson.class);
SingletonPerson bean2 = context.getBean(SingletonPerson.class);
assertThat(bean1).isEqualTo(bean2);
PrototypePerson bean3 = context.getBean(PrototypePerson.class);
PrototypePerson bean4 = context.getBean(PrototypePerson.class);
assertThat(bean3).isNotEqualTo(bean4);
}
@Scope
static class SingletonPerson {
private String name;
}
@Scope("prototype")
static class PrototypePerson {
private String name;
}
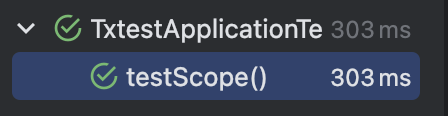
Web Aware Scope
The request scope creates a bean instance for a single HTTP request, while the session scope creates a bean instance for an HTTP Session.
테스트를 위한 Controller 코드
@Controller
@RequiredArgsConstructor
public class ScopeController {
private final ObjectProvider<MyLogger> myLoggerProvider;
private final LogService logService;
@GetMapping("/log-demo")
public String logDemo(HttpServletRequest request) throws InterruptedException {
final String requestURL = request.getRequestURL().toString();
final MyLogger myLogger = myLoggerProvider.getObject();
myLogger.setRequestUrl(requestURL);
myLogger.log("request scope test");
Thread.sleep(1000);
return "OK";
}
}
Request Scope
The ProxyMode attribute is necessary because at the moment of the instantion of the web application context, there is no active request.
Spring creates a proxy to be injected as a dependecy, and instantiates the target bean when it is needed in a request.
프록시 모드는 요청이 없는 경우 웹 애플리케이션 컨텍스트의 인스턴스화 상황 때문에 필요합니다.
요청이 생길 때 타겟 빈이 인스턴스화되어 사용됩니다.
Request Scope는 HTTP 요청마다 새로운 빈을 생성하여 사용합니다.
@Component
@Setter
@Scope("request")
public class MyLogger {
private String uuid;
private String requestUrl;
public void log(String msg) {
System.out.println("[" + uuid + "]" + "[" + requestUrl + "]" + msg);
}
@PostConstruct
public void init() {
uuid = UUID.randomUUID().toString();
System.out.println("[" + uuid + "] request scope bean created : " + this);
}
@PreDestroy
public void close() {
System.out.println("[" + uuid + "] request scope bean closed : " + this);
}
}

Session Scope
we need to run two requests in order to show that the value of the message field is the same for the session.
Session Sope 같은 경우는 하나의 세션 연결동안 생성한 빈을 세션 연결이 끊길 때까지 사용합니다.
@Component
@Setter
@Scope("session")
public class MyLogger {
private String uuid;
private String requestUrl;
public void log(String msg) {
System.out.println("[" + uuid + "]" + "[" + requestUrl + "]" + msg);
}
@PostConstruct
public void init() {
uuid = UUID.randomUUID().toString();
System.out.println("[" + uuid + "] request scope bean created : " + this);
}
@PreDestroy
public void close() {
System.out.println("[" + uuid + "] request scope bean closed : " + this);
}
}
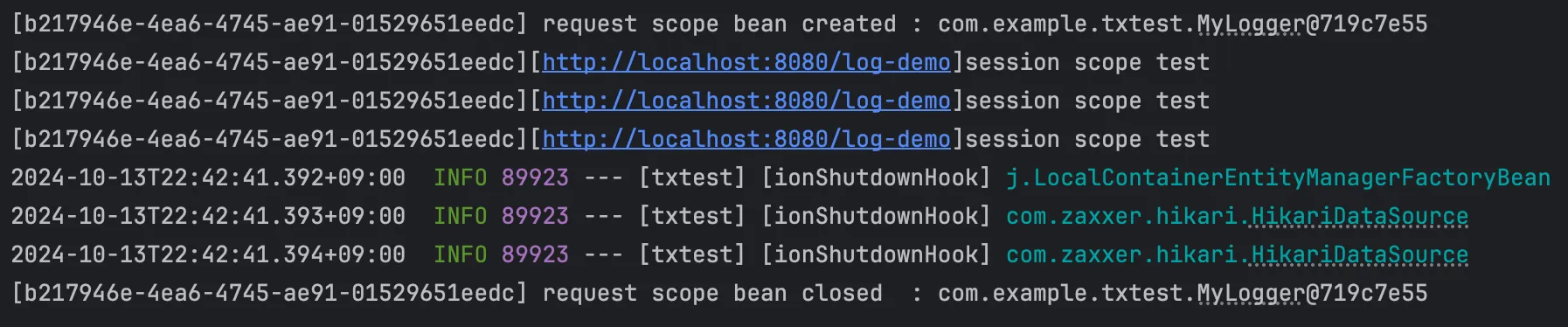
참고자료
Quick Guide to Spring Bean Scopes | Baeldung
Bean Scopes :: Spring Framework
7. [스프링 핵심 원리 - 기본편] 빈 스코프(Bean Scope)
'Spring Framework > Spring' 카테고리의 다른 글
Spring @RequestParam 페이징 정보 처리하기 - Pageable, @PageableDefault, sort (0) | 2024.10.19 |
---|---|
Spring StdSerializer, @JsonSerializer 커스텀 직렬화 처리 방법 (1) | 2024.10.16 |
스프링 FactoryBean: 생성자 주입과 필드 주입 시 프록시 객체의 동작 차이 (0) | 2024.10.12 |
Spring AOP 개념 정리 및 Aspect 적용 방법 - annotation 활용 (1) | 2024.10.10 |
[Spring] 스프링 FactoryBean 이해하기 - Custom Bean 생성방법 (2) | 2024.10.09 |