스프링 부트를 기반으로 테스트 코드 작성 시 자주 등장하는
테스트 어노테이션의 사용 상황과 기능에 대해 정리하고자 합니다.
@SpringBootTest 어노테이션
Annotation that can be specified on a test class that runs Spring Boot Based tests.
@SpringBootTest 어노테이션은 스프링 부트 기반 테스트 시에 사용하는 어노테이션이다.
다음과 같은 기능과 특징을 가지고 있습니다.
1. Uses SpringBootContextLoader as the default ContextLoader when no specific @ContextConfiguration(loader=...) is defined.
💬 특정 @ContextConfiguration을 정의하지 않는 이상, 기본적인 ContextLoader로는 SpringBootContextLoader를 사용합니다.
즉, 테스트 컨텍스트 초기화와 설정에 관한 역할을 수행합니다.
@SpringBootTest 간단 설명
- SpringBootContextLoader는 Springboot의 기본 설정을 로드하고, @SpringBootApplication, @Configuration 클래스 등을 기반으로 애플리케이션 컨텍스트를 초기화하는 어노테이션입니다.
@ContextConfiguration
@ContextConfiguration은 Springboot의 테스트 환경에서 특정 애플리케이션 컨텍스트를 로드하기 위해 사용합니다.
명시적으로 설정된 클래스를 기반으로 컨텍스트를 구성합니다.
모든 애플리케이션 컨텍스트가 아니라 특정 @Configuration을 로드할 때 사용합니다.
@TestConfiguration으로 설정한 빈
@TestConfiguration
public class AppConfig {
@Bean
public String message() {
return "Hello, ContextConfiguration!";
}
}
@ContextConfiguration을 통해 특정 빈 주입
// AppConfig 기반 컨텍스트 로드
@ContextConfiguration(classes = AppConfig.class)
public class AppConfigTest {
@Autowired
private String message;
@Test
public void testMessage() {
assertEquals("Hello, ContextConfiguration!", message);
}
}
2. Automatically searches for a @SpringBootConfiguration when nested @Configuration is not used, and no explicit classes are specified.
💬 @SpringBootTest 어노테이션은 중첩된 @Configuration 애플리케이션이 없는 이상, @SpringBootConfiguration를 자동으로 탐색합니다.
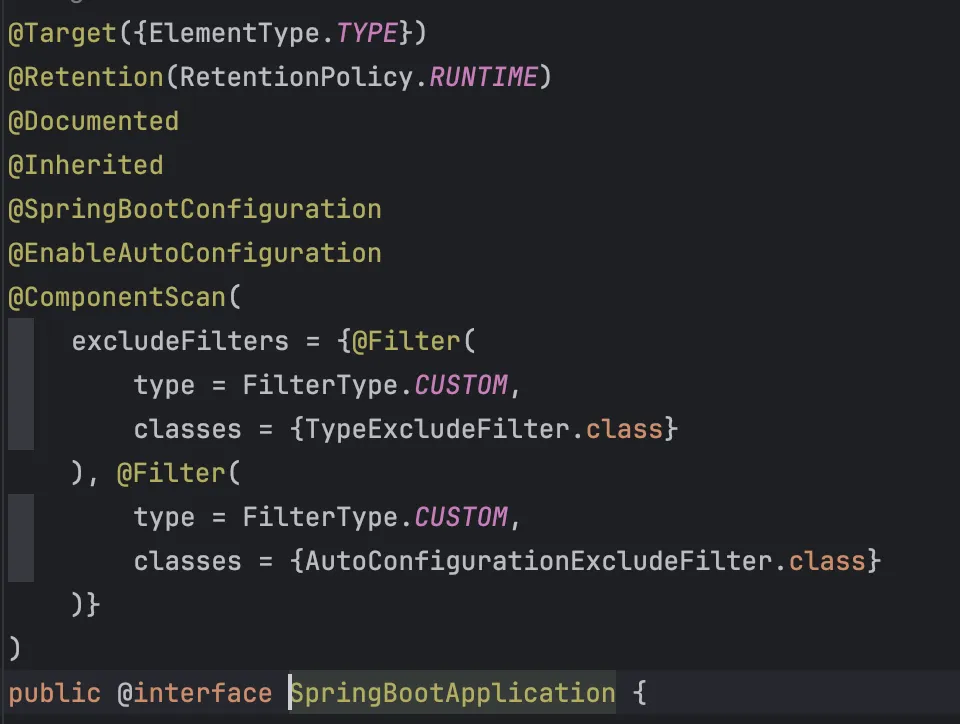
스프링부트 애플리케이션 메인 클래스에 포함되어 있는 어노테이션 내부에 있는 @SpringBootConfiguration을 최우선으로 탐색합니다. (주로 메인 클래스를 탐색함)
여기서 말하는 중첩 @Configuration이란 무엇인가?
테스트 환경에서 추가로 설정해줘야 하는 경우에 메인 환경과 테스트 환경에 같은 빈의 @Configuration이 존재할 수 있습니다. 테스트 컨텍스트를 직접 구성하기 위해 테스트 클래스 내부에 정의하는 자바 클래스를 의미합니다.
Nested Configuration 동작 원리
Spring Boot는 자동 설정의 일환으로 @SpringBootConfiguration을 탐지하여 애플리케이션 컨텍스트를 초기화합니다.
- 만약 @ContextConfiguration 또는 @SpringBootTest에 classes나 locations로 특정 설정을 지정하지 않았다면, Spring Boot는 클래스 경로에서 @SpringBootConfiguration이 선언된 클래스를 자동으로 찾습니다.
Nested @Configuration
@ContextConfiguration
public class CustomContextTest {
@Configuration // Nested @Configuration
static class TestConfig {
@Bean
public String testBean() {
return "Custom Bean";
}
}
@Autowired
private String testBean;
@Test
public void testCustomBean() {
assertEquals("Custom Bean", testBean);
}
}
위 코드 같은 경우 @Configuration이 제공되었으므로, SpringBoot는 @SpringBootConfiguration을 탐지하지 않고, 내부에 있는 TestConfig를 컨텍스트에 로드합니다.
번외
<김영한님>
📌
@SpringBootTest를 사용하면서, 그 안에서 내부 클래스로 @Configuration을 사용하게 되면 스프링 부트의 다양한 설정들이 먹히지 않고, @Configuration 안에서만 적용한 설정이 먹히게 됩니다.
→ nested @Configuration으로 테스트 클래스에서 재설정한 @Configuration이 적용된다. 이 설정이 우선권을 가지고 가버리기 때문에 스프링 부트의 다른 설정이 적용되지 않습니다.
@TestConfiguration을 사용하는 경우
스프링 부트가 제공하는 Transactional AOP 같은 기능들을 사용하려면 스프링 부트가 추가적인 빈들을 더 등록해야 합니다. @Configuration만 사용하면 트랜잭션이 AOP가 적용되지 않아서 오류가 발생합니다. 이럴 때 사용하는 것이 @TestConfiguration입니다.
@SpringBootTest를 사용하면서 @TestConfiguration을 사용하면 스프링 부트가 제공하는 기능들이 다 적용되고, 추가로 내가 등록한 빈들을 등록하여 사용할 수 있습니다.
이번 예제에서 Config 클래스에 @Configuration 을... - 인프런 | 커뮤니티 질문&답변
누구나 함께하는 인프런 커뮤니티. 모르면 묻고, 해답을 찾아보세요.
www.inflearn.com
@TestConfiguration
@SpringBootTest를 사용하면서 해당 어노테이션을 통해 스프링 부트가 제공하는 기능들과 추가로 내가 등록한 빈들을 등록하여 사용할 수 있는 테스트 어노테이션이다.
@WebMvcTest
Annotation that can be used for a Spring MVC test that focuses only on Spring MVC components.
Using this annotation only enables auto-configuration that is relevant to MVC tests. Simliarly, component scanning is limited to beans annotated with @Controller, @ControllerAdvice, @JsonComponent
💬 @WebMvcTest는 MVC 테스트 전용 테스트 어노테이션이다. (controller 계층 테스트용 어노테이션이라 생각할 수 있다.)
💬 mvc 테스트와 관련된 어노테이션만 자동-로드되는데, 로드되는 어노테이션은 @Controller, @ControllerAdvice, @JsonComponent .. 등 아래 사진과 같다.
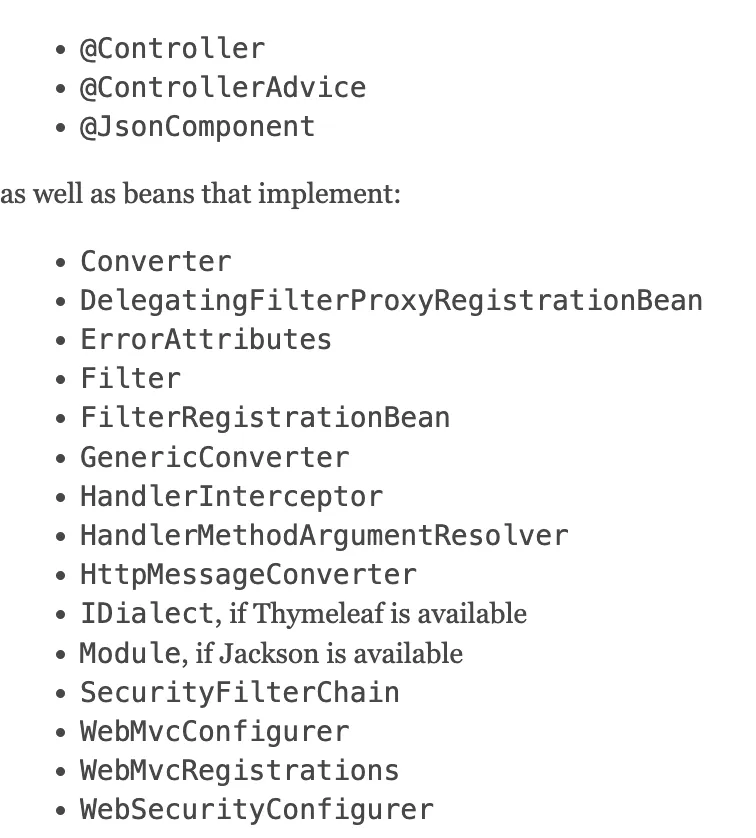
간단하게 Controller 즉 표현 계층과 관련된 설정들은 모두 로드된다고 볼 수 있다.
By default, tests annotated with @WebMvcTest will also auto-configure Spring Security and [MockMvc]
For more fine-grained control of MockMVC the @AutoConfigureMockMvc annotation can be used.
💬 @WebMvcTest는 스프링 시큐리티와 MockMvc를 자동 로드해 줍니다.
💬 MockMvc를 사용할 수 있는 또 다른 방법으로는 @AutoConfigurationMockMvc 어노테이션을 사용할 수 있습니다.
@WebMvcTest is used in combination with @MockBean or @Import to create any collaborators required by your @Controller beans.
💬 @webmvctest는 @mockbean, @import가 결합되어 사용됩니다.
테스트하려는 컨트롤러 빈(웹 계층)과 관련된 빈들을 생성해 줍니다.
If you are looking to load your full application configuration and use MockMVC, you should consider @SpringBootTest combined with @AutoConfigureMockMvc rather than this annotation
💬 만약, 더 큰 애플리케이션 환경에서 MockMvc를 사용하고 싶다면, @webmvctest가 아닌, @Springbootest와 @AutoConfigureMockMvc를 같이 사용해 처리할 수 있습니다.
@AutoConfigureMockMvc
Annotation that can be applied to a test class to enable and configure auto-configuration of MockMvc.
If AssertJ is available a MockMvcTester is auto-configured as well.
@WebMvcTest 어노테이션을 사용하지 않고, MockMvc를 사용하기 위해 테스트 클래스에서 사용할 수 있습니다.
(필터 설정과 같은 다른 기능들도 있습니다. 웹 계층 통합 테스트에 사용하기 적합함)
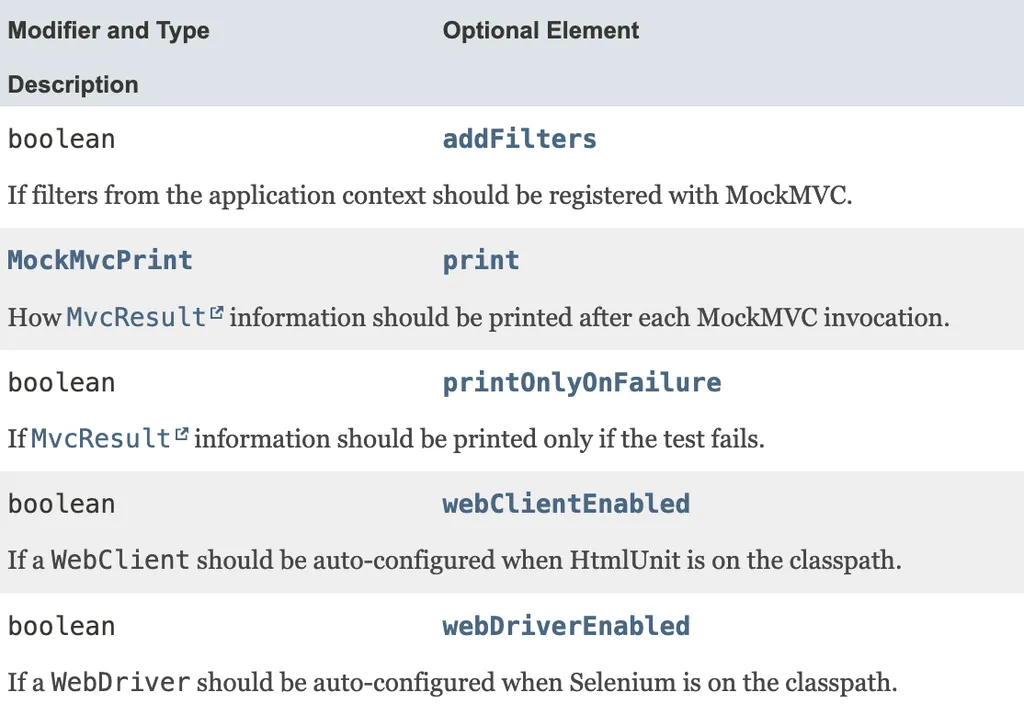
'Spring Framework > Spring boot' 카테고리의 다른 글
Spring BeanFactoryPostProcessor로 글로벌 @Lazy 설정 및 Bean 초기화하기 (0) | 2025.01.21 |
---|---|
Spring Boot @Lazy를 사용한 Bean 지연 초기화하기 (0) | 2025.01.21 |
Spring Boot Open-In-View 설정과 데이터베이스 성능 최적화, 영속성 컨텍스트 활용법 (1) | 2024.10.26 |
[SpringBoot] Spring에서 @Transactional과 try-catch 사용 시 롤백되지 않는 이유 (0) | 2024.10.09 |
[Spring] H2 In-memory 데이터베이스 설정 및 접속 방법 (3) | 2024.10.05 |
스프링 부트를 기반으로 테스트 코드 작성 시 자주 등장하는
테스트 어노테이션의 사용 상황과 기능에 대해 정리하고자 합니다.
@SpringBootTest 어노테이션
Annotation that can be specified on a test class that runs Spring Boot Based tests.
@SpringBootTest 어노테이션은 스프링 부트 기반 테스트 시에 사용하는 어노테이션이다.
다음과 같은 기능과 특징을 가지고 있습니다.
1. Uses SpringBootContextLoader as the default ContextLoader when no specific @ContextConfiguration(loader=...) is defined.
💬 특정 @ContextConfiguration을 정의하지 않는 이상, 기본적인 ContextLoader로는 SpringBootContextLoader를 사용합니다.
즉, 테스트 컨텍스트 초기화와 설정에 관한 역할을 수행합니다.
@SpringBootTest 간단 설명
- SpringBootContextLoader는 Springboot의 기본 설정을 로드하고, @SpringBootApplication, @Configuration 클래스 등을 기반으로 애플리케이션 컨텍스트를 초기화하는 어노테이션입니다.
@ContextConfiguration
@ContextConfiguration은 Springboot의 테스트 환경에서 특정 애플리케이션 컨텍스트를 로드하기 위해 사용합니다.
명시적으로 설정된 클래스를 기반으로 컨텍스트를 구성합니다.
모든 애플리케이션 컨텍스트가 아니라 특정 @Configuration을 로드할 때 사용합니다.
@TestConfiguration으로 설정한 빈
@TestConfiguration
public class AppConfig {
@Bean
public String message() {
return "Hello, ContextConfiguration!";
}
}
@ContextConfiguration을 통해 특정 빈 주입
// AppConfig 기반 컨텍스트 로드
@ContextConfiguration(classes = AppConfig.class)
public class AppConfigTest {
@Autowired
private String message;
@Test
public void testMessage() {
assertEquals("Hello, ContextConfiguration!", message);
}
}
2. Automatically searches for a @SpringBootConfiguration when nested @Configuration is not used, and no explicit classes are specified.
💬 @SpringBootTest 어노테이션은 중첩된 @Configuration 애플리케이션이 없는 이상, @SpringBootConfiguration를 자동으로 탐색합니다.
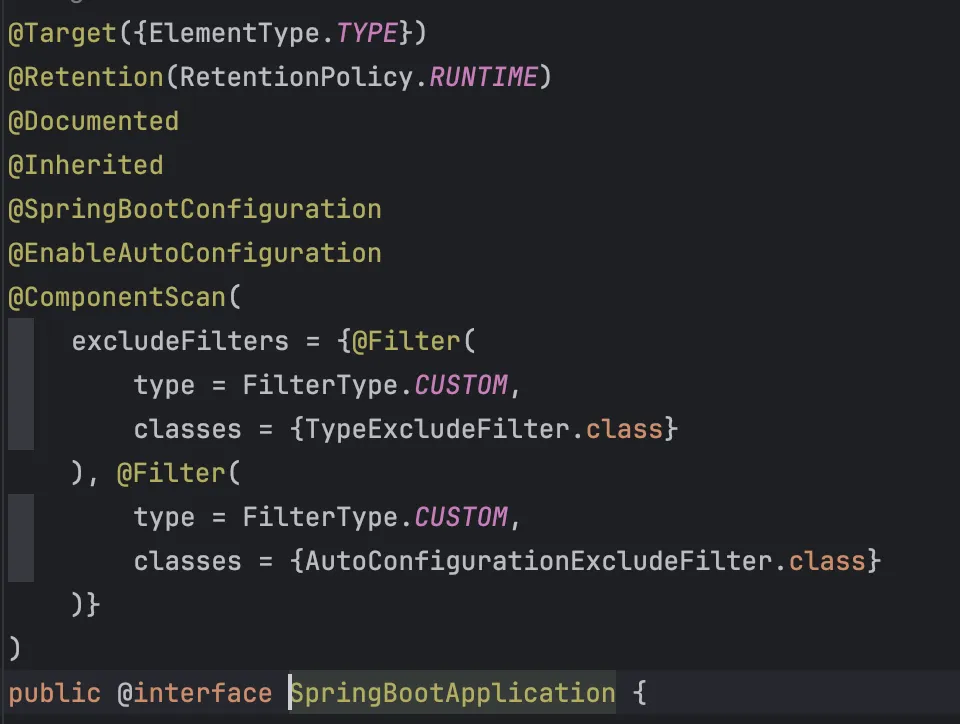
스프링부트 애플리케이션 메인 클래스에 포함되어 있는 어노테이션 내부에 있는 @SpringBootConfiguration을 최우선으로 탐색합니다. (주로 메인 클래스를 탐색함)
여기서 말하는 중첩 @Configuration이란 무엇인가?
테스트 환경에서 추가로 설정해줘야 하는 경우에 메인 환경과 테스트 환경에 같은 빈의 @Configuration이 존재할 수 있습니다. 테스트 컨텍스트를 직접 구성하기 위해 테스트 클래스 내부에 정의하는 자바 클래스를 의미합니다.
Nested Configuration 동작 원리
Spring Boot는 자동 설정의 일환으로 @SpringBootConfiguration을 탐지하여 애플리케이션 컨텍스트를 초기화합니다.
- 만약 @ContextConfiguration 또는 @SpringBootTest에 classes나 locations로 특정 설정을 지정하지 않았다면, Spring Boot는 클래스 경로에서 @SpringBootConfiguration이 선언된 클래스를 자동으로 찾습니다.
Nested @Configuration
@ContextConfiguration
public class CustomContextTest {
@Configuration // Nested @Configuration
static class TestConfig {
@Bean
public String testBean() {
return "Custom Bean";
}
}
@Autowired
private String testBean;
@Test
public void testCustomBean() {
assertEquals("Custom Bean", testBean);
}
}
위 코드 같은 경우 @Configuration이 제공되었으므로, SpringBoot는 @SpringBootConfiguration을 탐지하지 않고, 내부에 있는 TestConfig를 컨텍스트에 로드합니다.
번외
<김영한님>
📌
@SpringBootTest를 사용하면서, 그 안에서 내부 클래스로 @Configuration을 사용하게 되면 스프링 부트의 다양한 설정들이 먹히지 않고, @Configuration 안에서만 적용한 설정이 먹히게 됩니다.
→ nested @Configuration으로 테스트 클래스에서 재설정한 @Configuration이 적용된다. 이 설정이 우선권을 가지고 가버리기 때문에 스프링 부트의 다른 설정이 적용되지 않습니다.
@TestConfiguration을 사용하는 경우
스프링 부트가 제공하는 Transactional AOP 같은 기능들을 사용하려면 스프링 부트가 추가적인 빈들을 더 등록해야 합니다. @Configuration만 사용하면 트랜잭션이 AOP가 적용되지 않아서 오류가 발생합니다. 이럴 때 사용하는 것이 @TestConfiguration입니다.
@SpringBootTest를 사용하면서 @TestConfiguration을 사용하면 스프링 부트가 제공하는 기능들이 다 적용되고, 추가로 내가 등록한 빈들을 등록하여 사용할 수 있습니다.
이번 예제에서 Config 클래스에 @Configuration 을... - 인프런 | 커뮤니티 질문&답변
누구나 함께하는 인프런 커뮤니티. 모르면 묻고, 해답을 찾아보세요.
www.inflearn.com
@TestConfiguration
@SpringBootTest를 사용하면서 해당 어노테이션을 통해 스프링 부트가 제공하는 기능들과 추가로 내가 등록한 빈들을 등록하여 사용할 수 있는 테스트 어노테이션이다.
@WebMvcTest
Annotation that can be used for a Spring MVC test that focuses only on Spring MVC components.
Using this annotation only enables auto-configuration that is relevant to MVC tests. Simliarly, component scanning is limited to beans annotated with @Controller, @ControllerAdvice, @JsonComponent
💬 @WebMvcTest는 MVC 테스트 전용 테스트 어노테이션이다. (controller 계층 테스트용 어노테이션이라 생각할 수 있다.)
💬 mvc 테스트와 관련된 어노테이션만 자동-로드되는데, 로드되는 어노테이션은 @Controller, @ControllerAdvice, @JsonComponent .. 등 아래 사진과 같다.
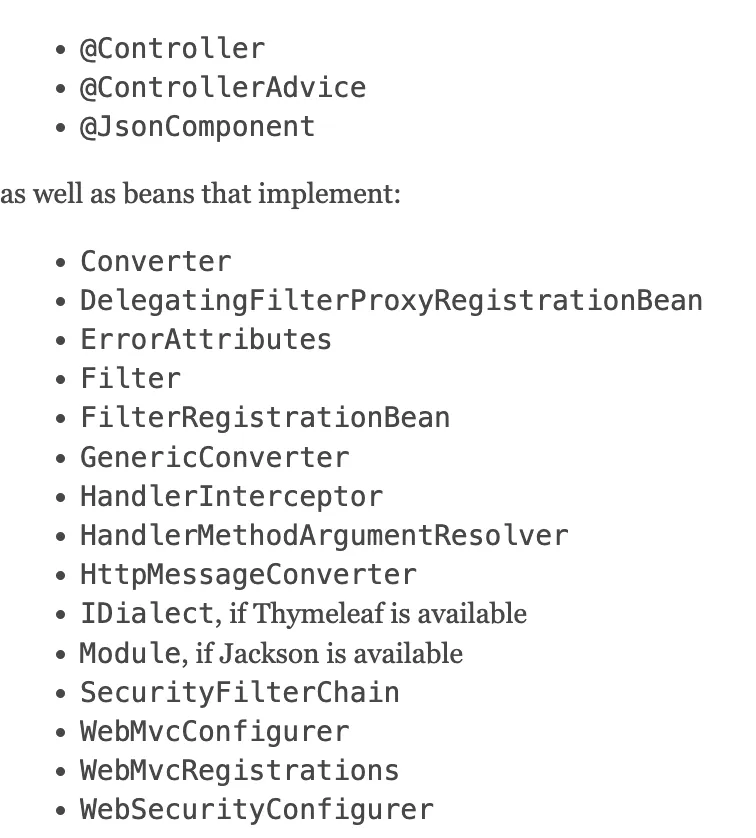
간단하게 Controller 즉 표현 계층과 관련된 설정들은 모두 로드된다고 볼 수 있다.
By default, tests annotated with @WebMvcTest will also auto-configure Spring Security and [MockMvc]
For more fine-grained control of MockMVC the @AutoConfigureMockMvc annotation can be used.
💬 @WebMvcTest는 스프링 시큐리티와 MockMvc를 자동 로드해 줍니다.
💬 MockMvc를 사용할 수 있는 또 다른 방법으로는 @AutoConfigurationMockMvc 어노테이션을 사용할 수 있습니다.
@WebMvcTest is used in combination with @MockBean or @Import to create any collaborators required by your @Controller beans.
💬 @webmvctest는 @mockbean, @import가 결합되어 사용됩니다.
테스트하려는 컨트롤러 빈(웹 계층)과 관련된 빈들을 생성해 줍니다.
If you are looking to load your full application configuration and use MockMVC, you should consider @SpringBootTest combined with @AutoConfigureMockMvc rather than this annotation
💬 만약, 더 큰 애플리케이션 환경에서 MockMvc를 사용하고 싶다면, @webmvctest가 아닌, @Springbootest와 @AutoConfigureMockMvc를 같이 사용해 처리할 수 있습니다.
@AutoConfigureMockMvc
Annotation that can be applied to a test class to enable and configure auto-configuration of MockMvc.
If AssertJ is available a MockMvcTester is auto-configured as well.
@WebMvcTest 어노테이션을 사용하지 않고, MockMvc를 사용하기 위해 테스트 클래스에서 사용할 수 있습니다.
(필터 설정과 같은 다른 기능들도 있습니다. 웹 계층 통합 테스트에 사용하기 적합함)
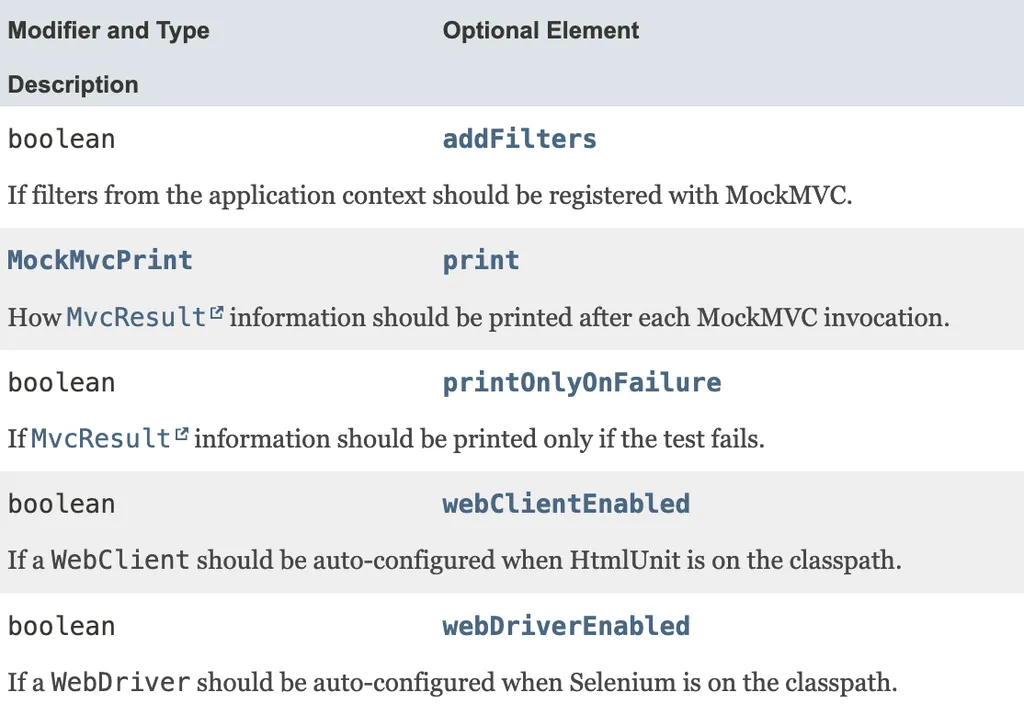
'Spring Framework > Spring boot' 카테고리의 다른 글
Spring BeanFactoryPostProcessor로 글로벌 @Lazy 설정 및 Bean 초기화하기 (0) | 2025.01.21 |
---|---|
Spring Boot @Lazy를 사용한 Bean 지연 초기화하기 (0) | 2025.01.21 |
Spring Boot Open-In-View 설정과 데이터베이스 성능 최적화, 영속성 컨텍스트 활용법 (1) | 2024.10.26 |
[SpringBoot] Spring에서 @Transactional과 try-catch 사용 시 롤백되지 않는 이유 (0) | 2024.10.09 |
[Spring] H2 In-memory 데이터베이스 설정 및 접속 방법 (3) | 2024.10.05 |